Concurrency and Multithreading: A Deep Dive
Concurrency and Multithreading: A Deep Dive
============================================
In the modern world of software development, concurrency and multithreading have become essential concepts to understand and master. As the demand for faster and more efficient applications grows, developers must learn how to effectively utilize these techniques to optimize their code and improve overall performance. In this article, we will take a deep dive into the world of concurrency and multithreading, exploring their definitions, differences, benefits, challenges, and best practices.
Table of Contents
-----------------
1. Introduction to Concurrency and Multithreading
2. Definitions and Concepts
- Process vs. Thread
- Context Switching
- Synchronization
- Deadlock
3. Benefits of Concurrency and Multithreading
4. Challenges and Drawbacks
5. Best Practices for Concurrency and Multithreading
6. Real-world Examples
7. Conclusion
1. Introduction to Concurrency and Multithreading
Concurrency and multithreading are two fundamental concepts in computer science that deal with the execution of multiple tasks simultaneously. Concurrency refers to the ability of a system to execute multiple tasks at the same time, while multithreading involves the execution of multiple threads within a single process.
Advertisement
2. Definitions and Concepts
# Process vs. Thread
A process is an instance of a running program in a computer system. It consists of the program code, data, and the resources required for execution, such as memory and I/O handles. A thread, on the other hand, is a smaller unit of execution within a process. Multiple threads can exist within a single process, allowing for parallel execution of tasks.
# Context Switching
Context switching is the process of storing and restoring the state of a process or thread so that execution can be resumed later. When a context switch occurs, the operating system saves the current state of the process or thread, including its registers and program counter, and restores the state of the next process or thread to be executed.
# Synchronization
Synchronization is the process of coordinating the execution of multiple threads to ensure that shared resources are accessed in a controlled manner. This is important to prevent race conditions and ensure data consistency. Synchronization can be achieved through various mechanisms, such as mutexes, semaphores, and monitors.
# Deadlock
A deadlock is a situation where two or more processes or threads are waiting indefinitely for resources held by each other, resulting in a standstill. Deadlocks can occur when multiple threads are accessing shared resources without proper synchronization, leading to a cyclic dependency.
3. Benefits of Concurrency and Multithreading
There are several benefits to using concurrency and multithreading in software development:
1. **Improved Performance**: By executing multiple tasks simultaneously, concurrency and multithreading can significantly improve the performance of applications, especially on multi-core processors.
2. **Responsiveness**: Multithreading allows applications to remain responsive to user input while performing background tasks, enhancing the overall user experience.
3. **Resource Utilization**: Concurrency enables better utilization of system resources, such as CPU and memory, by efficiently distributing tasks across available resources.
4. **Scalability**: Concurrency and multithreading can improve the scalability of applications, allowing them to handle increased workloads more effectively.
4. Challenges and Drawbacks
While concurrency and multithreading offer numerous benefits, they also come with their own set of challenges and drawbacks:
1. **Complexity**: Implementing concurrency and multithreading can increase the complexity of an application, making it more difficult to design, develop, and maintain.
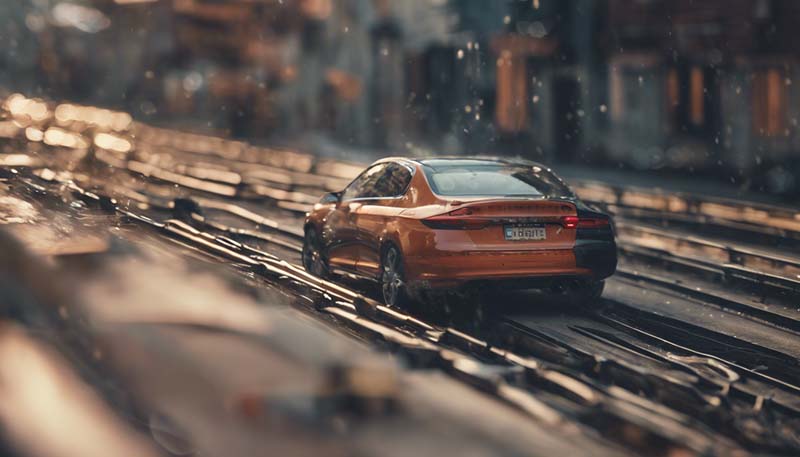
2. **Debugging**: Debugging concurrent and multithreaded applications can be challenging due to the non-deterministic nature of thread execution and potential race conditions.
3. **Race Conditions**: Race conditions occur when multiple threads access shared resources simultaneously, leading to unpredictable results and potential data corruption.
4. **Deadlocks**: As mentioned earlier, deadlocks can occur when threads are waiting indefinitely for resources held by each other, causing the application to become unresponsive.
5. Best Practices for Concurrency and Multithreading
To effectively utilize concurrency and multithreading in your applications, it\'s essential to follow best practices:
1. **Minimize Shared Resources**: Reduce the use of shared resources to minimize the risk of race conditions and deadlocks.
2. **Use Synchronization Mechanisms**: Employ synchronization mechanisms, such as mutexes, semaphores, and monitors, to control access to shared resources.
3. **Avoid Long-Running Tasks**: Long-running tasks can block other threads, causing performance issues. Break down long tasks into smaller, more manageable pieces.
4. **Leverage Concurrency Libraries**: Utilize existing concurrency libraries and frameworks, such as Java\'s java.util.concurrent package or C++\'s std::thread, to simplify the implementation of concurrent and multithreaded applications.
5. **Test Thoroughly**: Test your concurrent and multithreaded applications extensively to identify and resolve potential issues, such as race conditions and deadlocks.
6. Real-world Examples
Concurrency and multithreading are used in various real-world applications, including:
1. **Web Servers**: Web servers use multithreading to handle multiple client requests simultaneously, improving performance and responsiveness.
2. **Database Systems**: Database systems employ concurrency control mechanisms to ensure data consistency and integrity when multiple transactions are executed concurrently.
3. **Video Editing Software**: Video editing software often uses multithreading to perform complex video processing tasks, such as rendering and encoding, in parallel, reducing overall processing time.
4. **Scientific Computing**: Scientific applications, such as simulations and data analysis, can benefit from concurrency and multithreading by distributing computational tasks across multiple processors or cores.
7. Conclusion
Concurrency and multithreading are powerful techniques that can significantly enhance the performance and responsiveness of software applications. By understanding the concepts, benefits, challenges, and best practices associated with these techniques, developers can effectively utilize concurrency and multithreading to create efficient, scalable, and high-performing applications. However, it\'s crucial to approach concurrency and multithreading with caution, as they can introduce complexity and potential issues if not properly managed.