Efficient Code: Techniques for Writing Optimal Programs
Efficient Code: Techniques for Writing Optimal Programs
Writing efficient code is a skill that every programmer should strive to master. It's not just about making the code run faster, but also about making it more readable, maintainable, and scalable. In this article, we will explore various techniques that can help you write optimal programs.
Understanding Efficiency
Efficiency in code can be measured in terms of time complexity (how long it takes to run) and space complexity (how much memory it uses). The goal is to optimize both, but sometimes trade-offs are necessary.
Choosing the Right Data Structures
Using the right data structures is crucial for efficiency. For example, using a hash table (like a dictionary in Python or a map in C++) can provide O(1) access time, while a list would give O(n) access time.
Common Data Structures
- Arrays: Good for indexed access but inefficient for insertions and deletions.
- Linked Lists: Efficient for insertions and deletions but not for random access.
- Hash Tables: Provide fast access and insertion but can have issues with collisions.
- Trees: Useful for hierarchical data and can be optimized for search, insert, and delete operations.
Algorithmic Efficiency
Understanding the Big O notation is essential for assessing the efficiency of algorithms. Here are some common algorithmic paradigms:
Advertisement
Sorting Algorithms
Different sorting algorithms have different time complexities:
- Bubble Sort: O(n^2)
- Quick Sort: Average O(n log n), Worst O(n^2)
- Merge Sort: O(n log n)
Search Algorithms
Search algorithms also vary in efficiency:
- Linear Search: O(n)
- Binary Search: O(log n), but requires sorted input.
Code Optimization Techniques
Loop Unrolling
Loop unrolling is a technique where you manually write the body of a loop multiple times to reduce the overhead of loop control.
for (int i = 0; i < n; i += 5) {
process(i); process(i + 1); process(i + 2); process(i + 3); process(i + 4);
}
Avoiding Recomputation
Store the results of expensive function calls if the same inputs occur again.
int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
// Can be optimized using memoization
Inlining Functions
Inlining small, frequently called functions can reduce the overhead of function calls.
#define SQUARE(x) ((x) * (x))
Avoiding Premature Optimization
As Donald Knuth said, "Premature optimization is the root of all evil." Focus on writing clear code first, then optimize as needed.
Code Profiling and Analysis
Use profiling tools to identify bottlenecks in your code. Once you know where the inefficiencies are, you can focus your optimization efforts there.
Concurrency and Parallelism
Utilizing multiple cores or processors can greatly improve performance for certain types of tasks. Understand the difference between concurrency (dealing with a lot of things at once) and parallelism (doing a lot of things at once).
Memory Management
Understanding how memory is allocated and deallocated is crucial. Use smart pointers in C++ to manage memory automatically and avoid leaks.
Conclusion
Writing efficient code is a balance between speed, memory usage, readability, and maintainability. By understanding the principles of algorithmic complexity, choosing the right data structures, and using optimization techniques wisely, you can write programs that are both fast and easy to work with.
Remember, the most efficient code is the code that solves the problem with the least amount of work, and sometimes that means choosing simplicity over premature optimization.
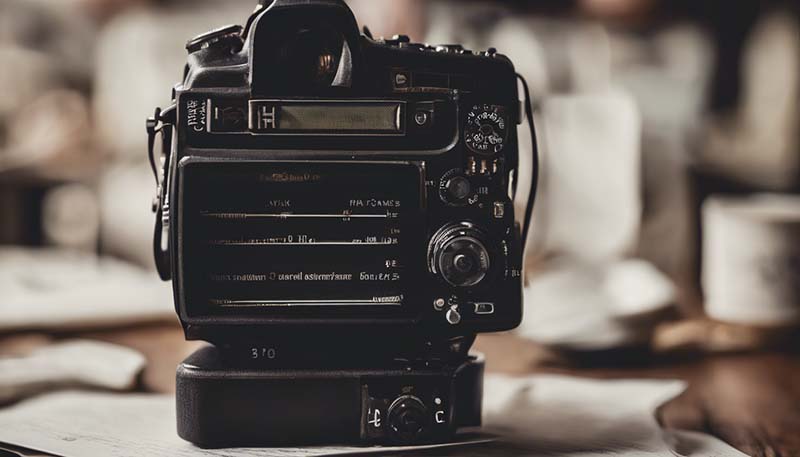