Design Patterns in Programming: Best Practices
Best Practices in Design Patterns
Introduction
Design patterns are reusable solutions to common problems in software design. They represent a set of best practices that the programmer can use to solve common issues when designing an application or system. In this article, we will explore various design patterns and discuss best practices for implementing them effectively.
Understanding Design Patterns
Design patterns are not complete solutions. Instead, they are templates for how to solve a problem in a specific context. They are high-level descriptions of common programming structures that can be used to solve common problems. Design patterns can be classified into three main categories:
- Creational Design Patterns
- Structural Design Patterns
- Behavioral Design Patterns
Creational Design Patterns
Creational design patterns provide object creation mechanisms that increase flexibility and reuse of existing code. They are responsible for object creation, and they abstract the instantiation process. Some common creational design patterns include:
- Singleton Pattern
- Factory Pattern
- Abstract Factory Pattern
- Builder Pattern
- Prototype Pattern
Structural Design Patterns
Structural design patterns explain how to assemble objects and classes into larger structures while keeping the structures flexible and efficient. They provide ways to compose objects into tree-like structures to represent part-whole hierarchies. Some common structural design patterns include:
Advertisement
- Adapter Pattern
- Bridge Pattern
- Composite Pattern
- Decorator Pattern
- Facade Pattern
- Flyweight Pattern
- Proxy Pattern
Behavioral Design Patterns
Behavioral design patterns are concerned with algorithms and the assignment of responsibilities between objects. They help to define the way objects and classes communicate and assign tasks to each other. Some common behavioral design patterns include:
- Chain of Responsibility Pattern
- Command Pattern
- Interpreter Pattern
- Iterator Pattern
- Mediator Pattern
- Memento Pattern
- Observer Pattern
- State Pattern
- Strategy Pattern
- Template Method Pattern
- Visitor Pattern
Best Practices for Implementing Design Patterns
When implementing design patterns, it is essential to follow best practices to ensure that the patterns are used effectively and do not introduce new problems. Here are some best practices to consider:
1. Know Your Patterns
Understanding the design patterns you are using is crucial. Know the purpose, structure, and implementation details of each pattern. This will help you to apply the pattern correctly and avoid misusing it.
2. Choose the Right Pattern
Carefully analyze the problem you are facing and choose the design pattern that best fits the problem. Do not force a pattern where it is not appropriate, as this can lead to a less flexible and more complex solution.
3. Keep It Simple
Design patterns should make your code more maintainable and understandable. Avoid overcomplicating your code by using too many patterns or using patterns that are too complex for the problem at hand.
4. Favor Composition Over Inheritance
Inheritance can lead to tight coupling and make your code less flexible. Favor composition over inheritance whenever possible, as it allows for more flexibility and easier maintenance.
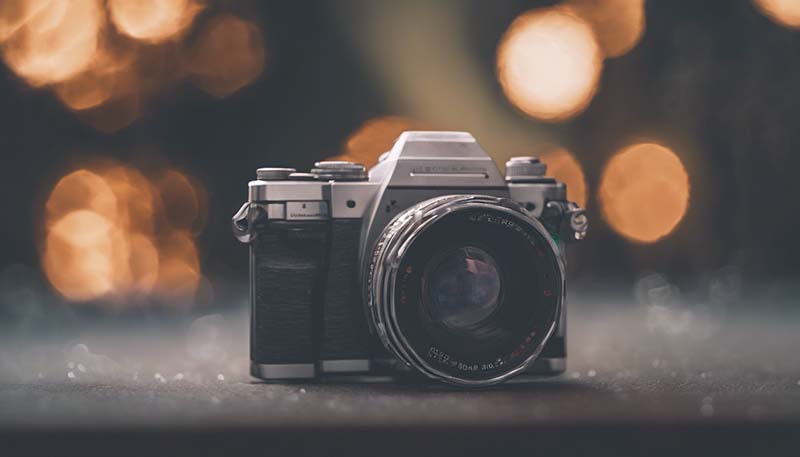
5. Document Your Design Decisions
When using design patterns, document your design decisions and the reasons why you chose a particular pattern. This will help other developers understand your choices and make it easier to maintain the code in the future.
6. Refactor with Care
Refactoring your code to use design patterns can improve its structure and maintainability. However, refactoring should be done with care to avoid introducing new bugs or making the code more complex than necessary.
7. Test Your Implementation
Testing is crucial when implementing design patterns. Ensure that your implementation of the pattern works as expected and that it does not introduce new bugs or issues.
8. Continuously Learn and Adapt
Design patterns are not a one-size-fits-all solution. Continuously learn about new patterns and best practices, and be willing to adapt your approach as you gain more experience and knowledge.
Conclusion
Design patterns are powerful tools that can help you create flexible, maintainable, and efficient software. By following best practices and understanding the patterns you are using, you can leverage these solutions to tackle common problems in software design effectively. Remember to keep your code simple, favor composition over inheritance, document your decisions, refactor with care, and continuously learn and adapt to make the most of design patterns in your programming projects.