Understanding Memory Management in Programming
Understanding Memory Management in Programming
Memory management is a critical aspect of programming that involves organizing and controlling how computer memory is allocated and used by a program. It is an essential concept for developers to understand, as it directly impacts the performance, efficiency, and stability of software applications. In this article, we will explore the fundamental concepts of memory management, its importance, various strategies, and common issues that programmers face.
Introduction to Memory Management
Computer memory, also known as RAM (Random Access Memory), is a temporary storage area used by the computer's processor to store and access data quickly. When a program is executed, it requires memory to store its instructions, data, and any intermediate results. Memory management is the process of allocating and deallocating memory for program execution efficiently and effectively.
Why is Memory Management Important?
Memory management is crucial for several reasons:
Advertisement
- Performance: Efficient memory management can significantly improve a program's performance by reducing the time spent on allocating and deallocating memory.
- Efficiency: Proper memory management prevents memory leaks and ensures that the program uses the minimum amount of memory necessary for its operation.
- Stability: Inadequate memory management can lead to program crashes, data corruption, and other stability issues.
- Security: Memory management can also impact the security of a program, as improper handling of memory can lead to vulnerabilities that can be exploited by attackers.
Memory Management Strategies
There are several strategies for managing memory in programming:
1. Manual Memory Management
In manual memory management, the programmer is responsible for allocating and deallocating memory explicitly. This approach provides the programmer with full control over memory usage but can be error-prone and lead to issues like memory leaks and dangling pointers.
2. Automatic Memory Management
Automatic memory management, also known as garbage collection, is a technique where the programming language runtime automatically manages memory allocation and deallocation. This approach reduces the risk of memory-related issues but can introduce performance overhead.
3. Pool Allocation
Pool allocation involves allocating a fixed-size pool of memory and managing the allocation of memory blocks within this pool. This strategy can be more efficient than manual memory management, as it reduces the overhead of allocating and deallocating small memory blocks.
4. Memory Mapping
Memory mapping is a technique used to map files or devices into the memory space of a program. This approach can be useful for working with large files or for sharing data between processes.
Common Memory Management Issues
Programmers often face several common issues related to memory management:
1. Memory Leaks
A memory leak occurs when a program allocates memory but fails to deallocate it when it is no longer needed. Over time, memory leaks can cause a program to consume increasing amounts of memory, leading to performance degradation and even program crashes.
2. Dangling Pointers
A dangling pointer is a pointer that references memory that has already been deallocated. Accessing memory through a dangling pointer can lead to unpredictable behavior and program crashes.
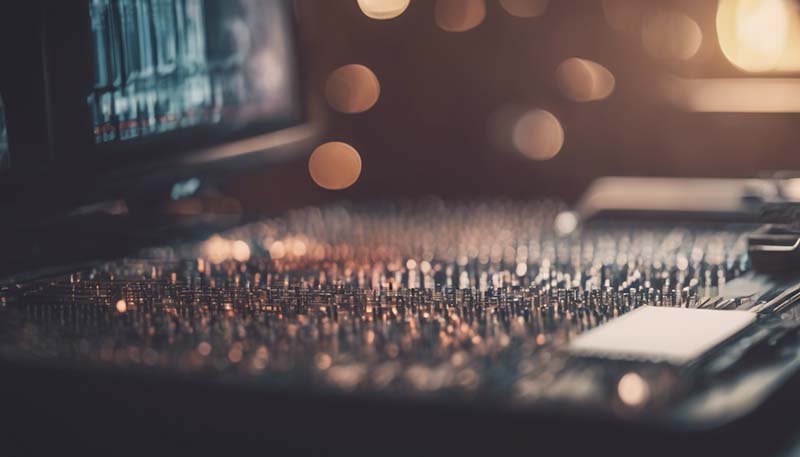
3. Buffer Overflows
A buffer overflow occurs when a program writes more data to a block of memory, or buffer, than it can hold. This can cause data to overwrite adjacent memory, potentially leading to security vulnerabilities and program instability.
4. Fragmentation
Memory fragmentation occurs when memory is allocated and deallocated in a way that leaves small, unusable blocks of memory scattered throughout the memory space. This can lead to inefficient use of memory and can cause performance issues.
Best Practices for Memory Management
To ensure effective memory management, programmers should follow these best practices:
- Understand the Memory Model: Familiarize yourself with the memory model of the programming language you are using, including how memory is allocated, accessed, and deallocated.
- Use Smart Pointers: In languages like C++, using smart pointers can help manage memory automatically and prevent issues like memory leaks and dangling pointers.
- Avoid Global Variables: Global variables can lead to unexpected memory usage and make it difficult to track memory allocation and deallocation.
- Profile Memory Usage: Regularly profile your program's memory usage to identify potential issues and areas for optimization.
- Handle Exceptions Safely: Ensure that your program properly handles exceptions and cleans up allocated memory even when exceptions occur.
Conclusion
Effective memory management is essential for building high-performance, stable, and secure software applications. By understanding the fundamental concepts, strategies, and best practices for memory management, developers can write more efficient and reliable code. As technology evolves and new programming languages and tools emerge, it is crucial to stay informed about the latest memory management techniques and best practices.