Understanding Recursion and Its Applications
Understanding Recursion and Its Applications
Introduction to Recursion
Recursion is a method of solving problems where the solution depends on solutions to smaller instances of the same problem. A recursive approach is one that can break down a complex problem into simpler, more manageable sub-problems. This technique is powerful and elegant but also can be confusing for those not familiar with it. Recursion is used in various fields, including computer science, mathematics, and linguistics.
Basic Concepts of Recursion
To understand recursion, you must grasp a few key concepts:
- Base Case: The base case is the condition under which the recursion ends. It's the simplest instance of the problem that can be solved directly without further recursion.
- Recursive Case: This is the part of the function that calls itself with a smaller or simpler input, moving towards the base case.
- Recursive Step: The step where the function's result is combined with other results to build towards the final answer.
- Stack Frame: Each recursive call adds a new frame to the call stack, which keeps track of local variables and the state of the function.
Recursive Function Example
Let's consider the classic example of calculating the factorial of a number using recursion:
Advertisement
def factorial(n): if n == 0: # Base case return 1 else: # Recursive case return n * factorial(n - 1) # Recursive step
Here, the base case is when n == 0
, and the recursive case is when n > 0
. The function calls itself with n - 1
.
Common Pitfalls of Recursion
While recursion is a powerful tool, it's not without its pitfalls:
- Infinite Recursion: If the base case is not properly defined or not reachable, the function can call itself indefinitely, leading to a stack overflow error.
- Performance Issues: Recursive functions can be less efficient than iterative solutions due to the overhead of multiple function calls and stack frames.
- Readability: While some recursive solutions can be more concise and easier to understand, others can become complex and difficult to follow.
Applications of Recursion
Recursion has a wide range of applications in computer science and other fields:
1. Algorithm Design
Many algorithms are more naturally expressed using recursion, such as:
- Tree Traversals (In-order, Pre-order, Post-order)
- Sorting Algorithms (Quicksort, Mergesort)
- Search Algorithms (Binary Search)
2. Divide and Conquer
The divide and conquer strategy involves breaking a problem into smaller sub-problems, solving each sub-problem recursively, and then combining the results. Recursion is a perfect fit for this approach.
3. Dynamic Programming
Recursion is often used to develop the recursive relations needed for dynamic programming, which are then used to build efficient algorithms that solve problems by solving sub-problems only once.
4. Backtracking
Backtracking is a form of recursion used to find all (or some) solutions to a problem, exploring every possible path and undoing steps that lead to dead ends.
5. Graph Theory
Recursion is used to solve problems related to graphs, such as finding paths, traversing, and searching for specific configurations.
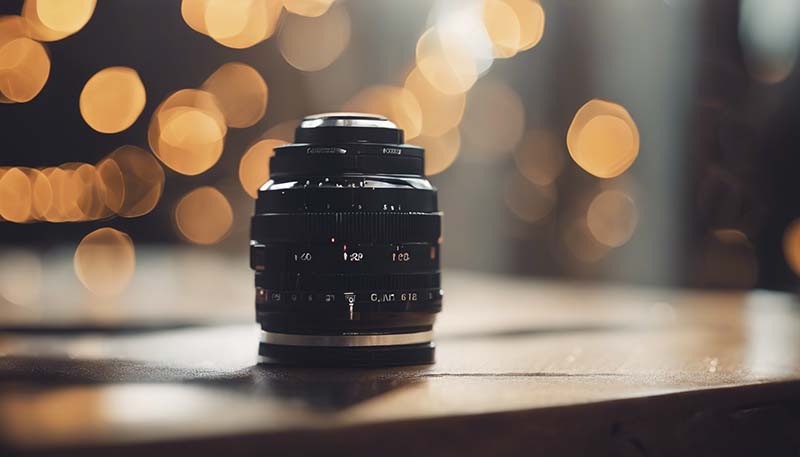
6. Artificial Intelligence and Machine Learning
In AI, recursion is used in various algorithms for searching through game trees (e.g., in chess or Go), solving puzzles, and optimizing decision-making processes.
7. Parsing and Computation
In compilers and interpreters, recursive descent parsing is a common technique for parsing expressions and statements in programming languages.
Recursive vs. Iterative Solutions
The choice between a recursive and an iterative solution often depends on the problem context, the language being used, and personal preference. Recursive solutions can be more elegant and easier to write, but iterative solutions can be more efficient in terms of memory and performance.
Conclusion
Recursion is a fundamental concept in computer science that offers a clear and concise way to solve complex problems. Understanding recursion requires practice and a solid grasp of the underlying principles. With the right approach, recursion can be a powerful tool in your programming arsenal.