Mastering Basic Math for Programming
Mastering Basic Math for Programming
Welcome to the essential guide on mastering basic math for programming. Mathematics forms a fundamental part of computer science and is crucial for understanding various programming concepts. This article covers the key mathematical topics that every programmer should know.
Table of Contents
- Introduction
- Arithmetic Operations
- Algebra
- Algorithms and Complexity
- Logic and Boolean Algebra
- Trigonometry
- Calculus
- Probability and Statistics
- Discrete Mathematics
- Additional Resources
Introduction
Mathematics is often seen as a barrier to entry for many aspiring programmers. However, understanding basic math concepts is not only helpful but necessary for solving complex problems and optimizing code. This guide aims to provide a solid foundation in the mathematical principles that are most relevant to programming.
Arithmetic Operations
Arithmetic is the most basic form of math and involves the study of numbers and basic operations such as addition, subtraction, multiplication, and division. In programming, arithmetic operations are fundamental for performing calculations and processing data.
Addition
Adding numbers is a common operation in programming, often used to aggregate data or calculate totals.
Subtraction
Subtraction is used to find differences between values, which can be essential in calculations like inventory management.
Advertisement
Multiplication
Multiplying numbers is used for scaling values, calculating areas, or volumes, and is a building block for more complex operations.
Division
Division is important when you need to split a value into equal parts or find an average.
Modulus
The modulus operation is used to find the remainder of a division operation, which is useful for determining the remainder in clock arithmetic or finding the remainder of a division.
Algebra
Algebra is the study of mathematical symbols and the rules for manipulating these symbols. It's a unifying thread of almost all of higher mathematics and includes everything from solving elementary equations to studying abstractions such as groups, rings, and fields.
Variables
In programming, variables are used to store values that can change. Understanding algebra helps in defining and manipulating variables in code.
Equations
Solving equations is a common task in programming, especially when dealing with problems that have a mathematical solution.
Functions
Algebraic functions are used to map input values to output values, a concept that is central to programming.
Algorithms and Complexity
Algorithms are step-by-step procedures for calculations. The complexity of an algorithm is often discussed in terms of its time and space complexity, which is closely related to the big O notation.
Logic and Boolean Algebra
Boolean algebra deals with binary variables and operations, which are integral to logic in programming. Logical operators such as AND, OR, and NOT are used to control the flow of programs and make decisions based on conditions.
Trigonometry
While not as commonly used as other areas of math, trigonometry can be important in fields like computer graphics, physics simulations, and any application that involves angles, waves, or oscillations.
Calculus
Calculus is the study of change, and it's used in programming for tasks that involve rates of change, areas, and volumes. It's essential in fields like artificial intelligence, game development, and engineering simulations.
Probability and Statistics
Probability theory and statistics are used in programming to analyze data, make predictions, and handle uncertainty. They are fundamental to machine learning, data science, and any application that involves randomness or data analysis.
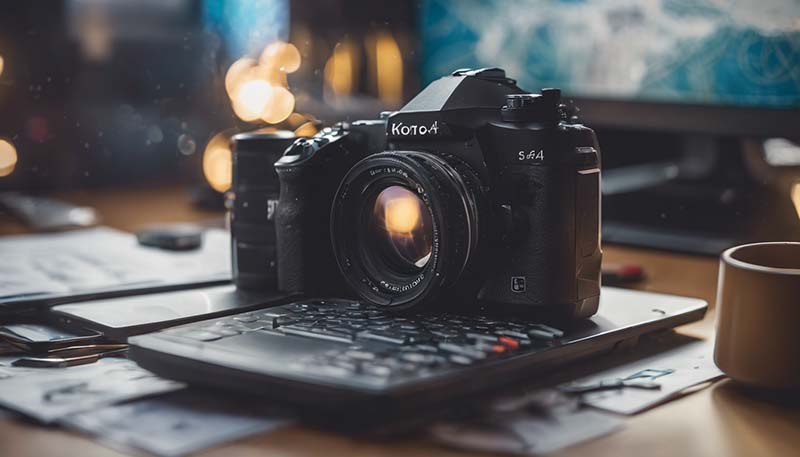
Discrete Mathematics
Discrete mathematics deals with separate, distinct values, and it is especially important in computer science. Topics like combinatorics, graph theory, and logic are all part of discrete mathematics and have direct applications in programming.
Additional Resources
For further study, here are some resources that can help deepen your understanding of the mathematical concepts related to programming:
- Khan Academy (<>) - Offers free courses on a wide range of mathematical topics.
- Coursera (<>) - Provides university-level courses in mathematics and computer science.
- MIT OpenCourseWare (<>) - Offers materials from MIT courses, including many on mathematics and computer science.
By mastering the basic math concepts outlined in this guide, you'll be better equipped to tackle a wide range of programming challenges and improve your overall problem-solving skills.