The Role of Functions in Modular Programming
Modular programming is a software design technique that emphasizes the division of a program into separate, interchangeable modules. Each module encapsulates a specific functionality or feature and can be developed, tested, and maintained independently. One of the key components of modular programming is the use of functions. Functions play a crucial role in modular programming by providing a way to organize code, promote code reuse, and improve code readability and maintainability.
## Understanding Functions
A function is a block of code that performs a specific task and can be called from other parts of the program. Functions can take input parameters, perform some operations on those parameters, and return a result. The basic structure of a function includes a function declaration, input parameters, and a return statement.
Function Declaration
A function declaration defines the name of the function, its input parameters, and the data type of the return value. For example, consider the following function declaration in C++:
Advertisement
cpp
int add(int a, int b) {
// Function body
}
In this example, the function add takes two integer parameters a and b and returns an integer value.
Input Parameters
Input parameters are the values passed to a function when it is called. They allow a function to accept different inputs and perform operations based on those inputs. Parameters can be of different data types, such as integers, strings, or custom data structures.
Return Statement
A return statement is used to specify the result of a function. It can be a single value or an expression that evaluates to a value. The return value can be used by other parts of the program or passed as an input parameter to another function.
## Benefits of Using Functions
Using functions in modular programming offers several benefits:
Code Organization
Functions provide a way to organize code into smaller, manageable units. By grouping related functionality into a single function, the code becomes easier to understand and maintain.
Code Reusability
Functions promote code reuse by allowing a piece of code to be executed multiple times with different input parameters. This eliminates the need to duplicate code and reduces the chances of introducing errors.
Improved Readability
Functions improve code readability by providing a clear, concise description of the code\'s purpose. This makes it easier for other developers to understand the code and reduces the time required to debug and troubleshoot issues.
Maintainability
Functions make code maintenance easier by isolating specific functionalities. If a bug or issue is found in a particular function, it can be fixed without affecting other parts of the program.
## Best Practices for Using Functions
To maximize the benefits of using functions in modular programming, follow these best practices:
Keep Functions Small and Focused
Functions should be small and focused, performing a single task or operation. This makes the code easier to understand and maintain.
Use Descriptive Function Names
Function names should be descriptive and accurately reflect the purpose of the function. This makes it easier for other developers to understand the code and reduces the chances of introducing errors.
Avoid Side Effects
Functions should be side-effect free, meaning they should not modify any external variables or state. This ensures that the function\'s behavior is predictable and makes it easier to test and debug.
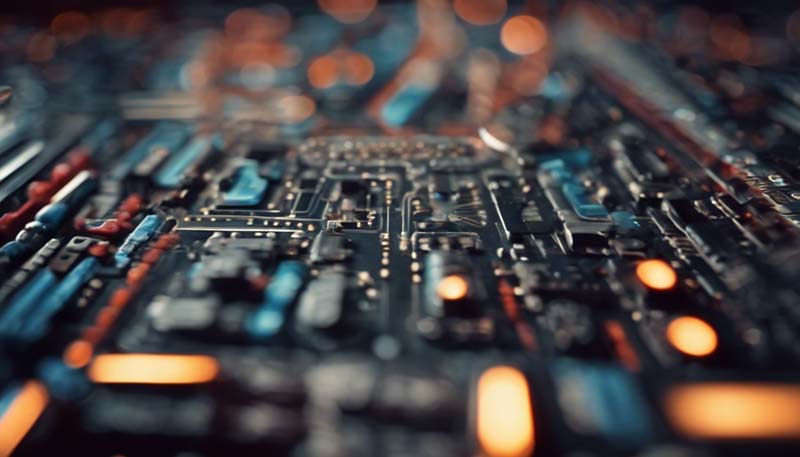
Use Input Parameters for Customization
Input parameters should be used to customize the behavior of a function. This allows the same function to be reused for different scenarios and reduces code duplication.
Return Values for Output
Return values should be used to communicate the result of a function. This allows the function\'s output to be used by other parts of the program or passed as an input parameter to another function.
## Conclusion
Functions play a crucial role in modular programming by providing a way to organize code, promote code reuse, and improve code readability and maintainability. By following best practices for using functions, developers can create modular, maintainable, and scalable software systems.