Loops and Iterations: Enhancing Efficiency in Code
Loops and Iterations: Enhancing Efficiency in Code
=======================================================================
Introduction
------------
In computer programming, loops and iterations are fundamental constructs that enable developers to execute a block of code repeatedly until a specific condition is met. They are essential for managing repetitive tasks, processing data, and implementing algorithms. Efficient use of loops can lead to significant improvements in code performance, readability, and maintainability. This article will explore various types of loops, their applications, and best practices for enhancing efficiency in code.
Why Use Loops?
1. **Repetitive Tasks**: Automate the execution of repetitive operations.
2. **Data Processing**: Iterate through arrays, lists, or other collections of data.
3. **Control Flow**: Implement complex control flows and conditions.
4. **Algorithm Implementation**: Many algorithms rely on loops for execution.
Types of Loops
1. **For Loop**: Iterates a specific number of times.
2. **While Loop**: Continues until a condition becomes false.
3. **Do-While Loop**: Executes at least once and continues if a condition is true.
Advertisement
4. **ForEach Loop**: Iterates through elements of a collection without the need for an index.
Enhancing Efficiency
To enhance efficiency in code, we must consider the following:
1. **Minimize Work Inside Loops**: Perform only necessary operations within the loop.
2. **Avoid Infinite Loops**: Always ensure there\'s a condition to break out of the loop.
3. **Use Loops Judiciously**: Choose the right type of loop for the task.
4. **Optimize Loop Control Variables**: Use appropriate data types and initializations.
5. **Break and Continue**: Use these statements to control loop flow effectively.
6. **Loop Unrolling**: Manually replicate loop body to reduce overhead in certain scenarios.
7. **Algorithmic Efficiency**: Choose algorithms with lower time complexity.
Understanding Loop Constructs
-------------------------------
For Loop
The for loop is commonly used when the number of iterations is known beforehand.
java
for (int i = 0; i < n; i++) {
// Code to execute
}
While Loop
The while loop is used when the number of iterations is not known and depends on a condition.
java
int i = 0;
while (i < n) {
// Code to execute
i++;
}
Do-While Loop
The do-while loop is similar to the while loop but ensures that the code block is executed at least once.
java
int i = 0;
do {
// Code to execute
i++;
} while (i < n);
ForEach Loop
The forEach loop is used to iterate through collections without using an index.
java
for (int number : numbers) {
// Code to execute with number
}
Loop Efficiency Techniques
-------------------------
Minimize Work Inside Loops
Every operation inside a loop can significantly impact performance if the loop iterates many times. It\'s crucial to minimize the work done inside the loop.
**Example:**
java
// Inefficient - performing unnecessary work inside the loop
for (int i = 0; i < largeNumber; i++) {
String s = String.valueOf(i);
// Other operations
}
// Efficient - perform heavy operations outside the loop
String[] sArray = new String[largeNumber];
for (int i = 0; i < largeNumber; i++) {
sArray[i] = String.valueOf(i);
}
Avoid Infinite Loops
Ensure that the loop has a clear exit condition to prevent infinite iterations.
**Example:**
java
// Infinite loop - missing break condition
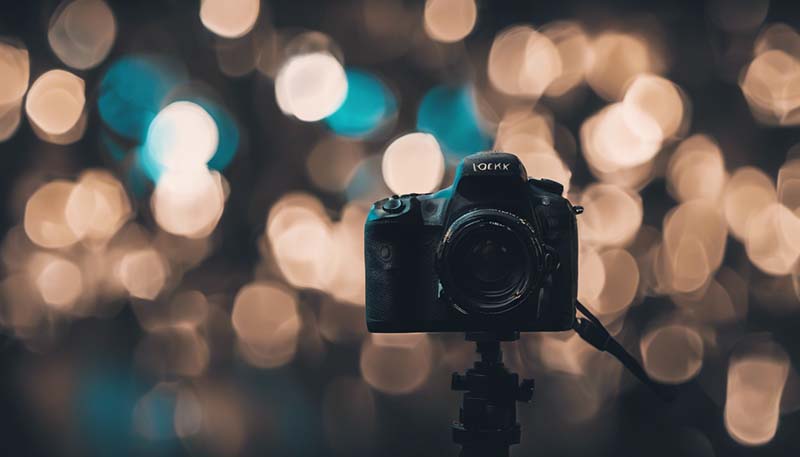
while (true) {
// Code to execute
}
// Finite loop with a break condition
int count = 0;
while (count < n) {
// Code to execute
count++;
}
Use Loops Judiciously
Select the appropriate loop type based on the task. For example, use a for loop when the number of iterations is fixed, and a while loop when the end condition is based on a dynamic state.
Optimize Loop Control Variables
Choose the correct data type for loop control variables to prevent unnecessary casting or type overflows.
**Example:**
java
// Using an int for a loop that won\'t exceed the int range is efficient
for (int i = 0; i < MAX_VALUE; i++) {
// Code to execute
}
// Using a long if the iteration count could exceed int\'s range
for (long i = 0; i < SOME_LARGE_VALUE; i++) {
// Code to execute
}
Break and Continue Statements
Use break to exit a loop prematurely when a condition is met, and continue to skip the current iteration and move to the next one.
**Example:**
java
for (int i = 0; i < numbers.length; i++) {
if (numbers[i] == 0) {
continue; // Skip processing for zero values
}
// Process numbers[i]
}
for (int i = 0; i < n; i++) {
if (someCondition) {
break; // Exit loop if condition is met
}
// Code to execute
}
Loop Unrolling
Loop unrolling is a technique where the loop body is manually replicated to reduce the overhead of loop control. It can be beneficial in certain scenarios but should be used with caution as it can make the code less readable.
**Example:**
java
// Unrolled loop
for (int i = 0; i < n; i += 2) {
// Process elements i and i+1
// ...
}
Algorithmic Efficiency
Always consider the algorithm\'s efficiency. For example, using a linear search in a sorted array when a binary search would be more efficient.
**Example:**
java
// Inefficient - linear search
for (int i = 0; i < sortedArray.length; i++) {
if (sortedArray[i] == target) {
return i; // Found the target
}
}
// Efficient - binary search (assuming the array is sorted)
// ...
Conclusion
----------
Loops and iterations are powerful tools in a programmer\'s arsenal. By understanding the different types of loops and applying best practices for efficiency, developers can write clean, performant, and maintainable code. Always profile your code to identify bottlenecks and consider the trade-offs when optimizing loops. Remember, readability and maintainability are also important, so avoid over-optimizing to the point where the code becomes difficult to understand or maintain.