Variables: The Building Blocks of Programming
Variables: The Building Blocks of Programming
Programming is a complex activity that involves creating instructions for a computer to follow. At the heart of programming are variables, which are the fundamental building blocks that allow us to store, manipulate, and communicate data within our programs. This article will explore the concept of variables, their importance, types, and how they are used in programming.
What is a Variable?
A variable is a named storage location in a computer's memory that can hold a value. The value of a variable can be changed throughout the execution of a program, which is why it's called a "variable" – it varies. Variables are a way for programmers to create a symbolic name for a memory location, making the code more readable and manageable.
Why are Variables Important?
Variables are essential for several reasons:
- Storing Data: Variables allow us to store data that can be used later in the program.
- Manipulating Data: We can perform operations on the data stored in variables, such as addition, subtraction, concatenation, etc.
- Memory Management: By using variables, we can manage the computer's memory more efficiently.
- Readability: Variables make the code more readable and easier to understand by giving meaningful names to data.
- Reusability: Variables can be reused throughout a program, which reduces redundancy and improves efficiency.
Types of Variables
Variables can be classified into several types based on their characteristics:
Advertisement
1. Scalar Variables
Scalar variables store a single value. The most common scalar variables are:
- Integers: Whole numbers, such as 1, 2, 3, etc.
- Floats: Numbers with decimal points, such as 3.14, -2.5, etc.
- Booleans: Logical values that can be either true or false.
- Characters: Single characters, such as 'A', 'b', etc.
2. Aggregate Variables
Aggregate variables store a collection of values. The main types of aggregate variables are:
- Arrays: An ordered collection of elements, all of the same type.
- Lists: Similar to arrays, but more flexible and can contain elements of different types.
- Structures: A collection of different types of variables grouped together.
- Objects: In object-oriented programming, objects are instances of classes and can contain data and methods.
3. Pointer Variables
Pointer variables store the memory address of another variable. They are used to directly access and manipulate the memory and are a powerful feature in many programming languages.
Declaring and Initializing Variables
To use a variable in a program, it must first be declared and then initialized. Declaration tells the compiler about the variable's type and name, while initialization assigns a value to the variable.
// Declaring and initializing a variable in C
int age = 30;
In the example above, `age` is a variable of type `int` (integer), and it's initialized with the value `30`.
Variable Scope and Lifetime
The scope of a variable determines where in the program it can be accessed and used. There are typically four types of scopes:
- Global Scope: Variables with global scope can be accessed from anywhere in the program.
- Local Scope: These variables are only accessible within the block (usually a function or a loop) they are defined in.
- Parameter Scope: Variables that are parameters of a function are only accessible within that function.
- Class Scope (in OOP): In object-oriented programming, variables can have class scope if they are defined within a class.
The lifetime of a variable refers to the duration for which the variable exists in memory. The lifetime depends on the scope and the type of the variable (static, stack, or heap).
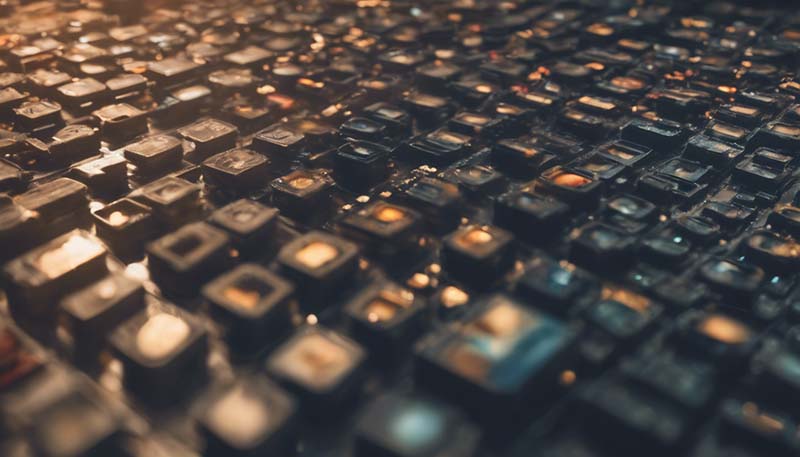
Best Practices for Using Variables
When using variables, it's important to follow best practices to write clean and efficient code:
- Meaningful Names: Use descriptive names for variables to make the code self-explanatory.
- Consistent Naming Conventions: Follow a consistent naming convention throughout the codebase.
- Initialization: Always initialize variables before using them to avoid undefined behavior.
- Avoid Global Variables: Minimize the use of global variables as they can lead to code that is difficult to maintain and debug.
- Use Constants for Unchanging Data: If a value doesn't change, consider using a constant instead of a variable.
Conclusion
Variables are a fundamental concept in programming, and understanding how to use them effectively is crucial for any programmer. By mastering the art of working with variables, you can write more efficient, readable, and maintainable code. Always remember to declare and initialize your variables carefully, choose meaningful names, and follow best practices to ensure your code is robust and easy to understand.